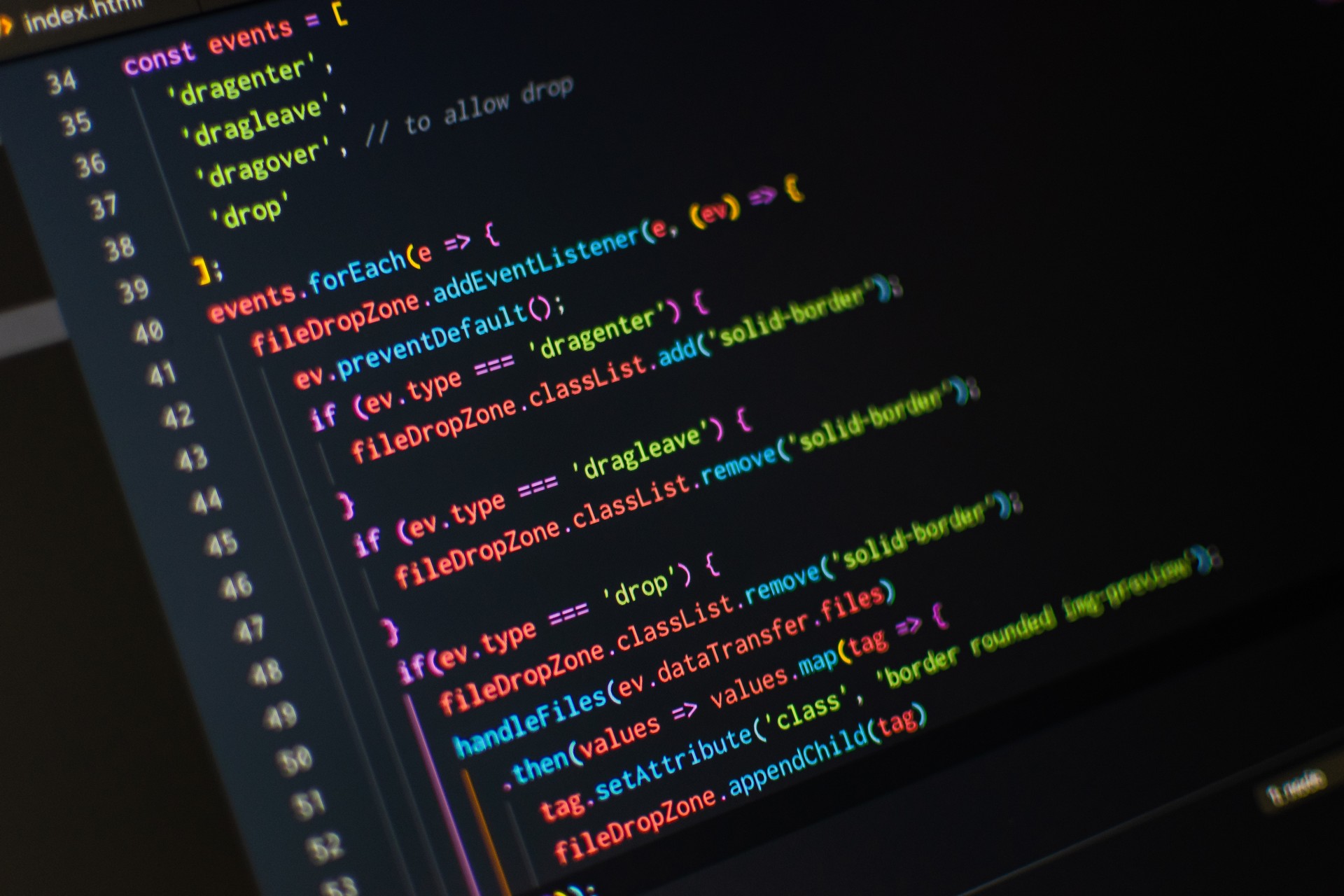
JavaScript tricks for every developer
With JavaScript, the most popular programming language for web development for both front-end and back-end, you can do (almost) anything these days. There are countless frameworks that allow you to write web apps quickly and easily. To make it even easier (read: clearer and more maintainable), I've listed some of my favorite tips & tricks here.
Arrow functions
1 2 3 4
const calculateAge = (birthYear) => new Date().getFullYear() - birthYear; calculateAge(1989);
Above you see an example of a function, but written as an arrow function. At first glance, except for a different syntax, it's no different from regular functions, so why choose this syntax over the traditional way of writing functions?
- This syntax ensures that the
this
is automatically bound to the surrounding code. - An implicit return is also provided when there is no body block which makes the code shorter and in most cases simpler.
- And of course, the
=>
notation is shorter and simpler than withfunction
(although this may be subjective).
Pass arguments as object
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
const displayAlbum = ({name, artist, tracks}) => { // display the album } displayAlbum({ name: 'Bat Out of Hell', artist: 'Meat Loaf', tracks: [ 'Bat Out of Hell', 'You Took the Words Right Out of My Mouth (Hot Summer Night)', // other tracks ] }); displayAlbum({ artist: 'Pink Floyd', name: 'The Dark Side of the Moon', tracks: [ 'Speak to Me', 'Breathe', // other tracks ] });
Instead of passing arguments one by one, you can also choose to do this in one object. This has some advantages:
- the order of the arguments no longer matters – note above that in the first call the order is
name
,artist
,tracks
, while in the second isartist
,name
,tracks
; - most IDEs will now provide more targeted information on the specific argument you pass;
- this communicates the intent in a clear way because function calls specify the value of each property;
- especially large codebases will benefit greatly from this.
Using Generators to determine sequential IDs
1 2 3 4 5 6 7 8 9 10 11 12 13 14
function* infinite() { let index = 0; while (true) { yield index++; } } const generator = infinite(); console.log(generator.next().value); // 0 console.log(generator.next().value); // 1 console.log(generator.next().value); // 2 // ...
With the introduction of generators in ES2015 (ES6), determining infinitely consecutive IDs has never been easier. These are functions that apply lazy evaluation by using yield
to process and return data.
At first sight it seems that the above generator will consume a lot of CPU over time, but nothing could be further from the truth. Generators describe a state mechanism where transitions can occur through the code. These transitions occur on-demand when the next method is called. Hence the term lazy evaluation.
The optional chaining operator
1 2 3 4 5 6 7 8 9 10 11
const album = { name: 'Bat Out of Hell', artist: 'Meat Loaf', onChangeCallback: () => { return { changed: true } } }; console.log(album?.name); // Bat Out of Hell console.log(album?.onChangeCallback?.()); // { changed: true } console.log(album?.onDeleteCallback?.()); // undefined
Now that the optional chaining operator is supported in almost all browsers, it is easier to parse complex objects. In the past, developers had to use if statements to check whether a particular variable or method existed before calling it. Now it's a lot easier, just by using a ?
. As you can see, you can even do a function call afterwards, by adding two parentheses after ?.
.
Destructuring
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
const album = { name: 'Bat Out of Hell', artist: 'Meat Loaf', tracks: [ 'Bat Out of Hell', 'You Took the Words Right Out of My Mouth (Hot Summer Night)', 'Heaven Can Wait' // other tracks ] }; const { name, artist } = album; const { 0: track1, 2: track3 } = album.tracks; console.log(name); console.log(artist); console.log(track1); console.log(track3);
Another simple trick is destructuring a JavaScript object. Here, data is extracted from an object and stored in separate variables, thus ensuring shorter and more readable lines of code in later code. This is certainly recommended with frameworks such as React, when you use a StyleSheet
or when complex props
come in.
Remove duplicates using Set
1 2 3 4 5 6 7 8 9 10 11
const albums = [ 'Thriller', 'Back in Black', 'Bat Out of Hell', 'The Dark Side of the Moon', 'Bat Out of Hell', 'Thriller' ]; const uniqueAlbums = [...new Set(albums)]; console.log(uniqueAlbums); // ['Thriller', 'Back in Black', 'Bat Out of Hell', 'The Dark Side of the Moon']
A very simple, but also very effective way to filter duplicates from an array is to transfer this array to a Set. A Set cannot contain duplicate values, so a duplicate value is only stored once in that Set. In the example above, an array of strings is filtered, but this can also be done with other types, including objects!