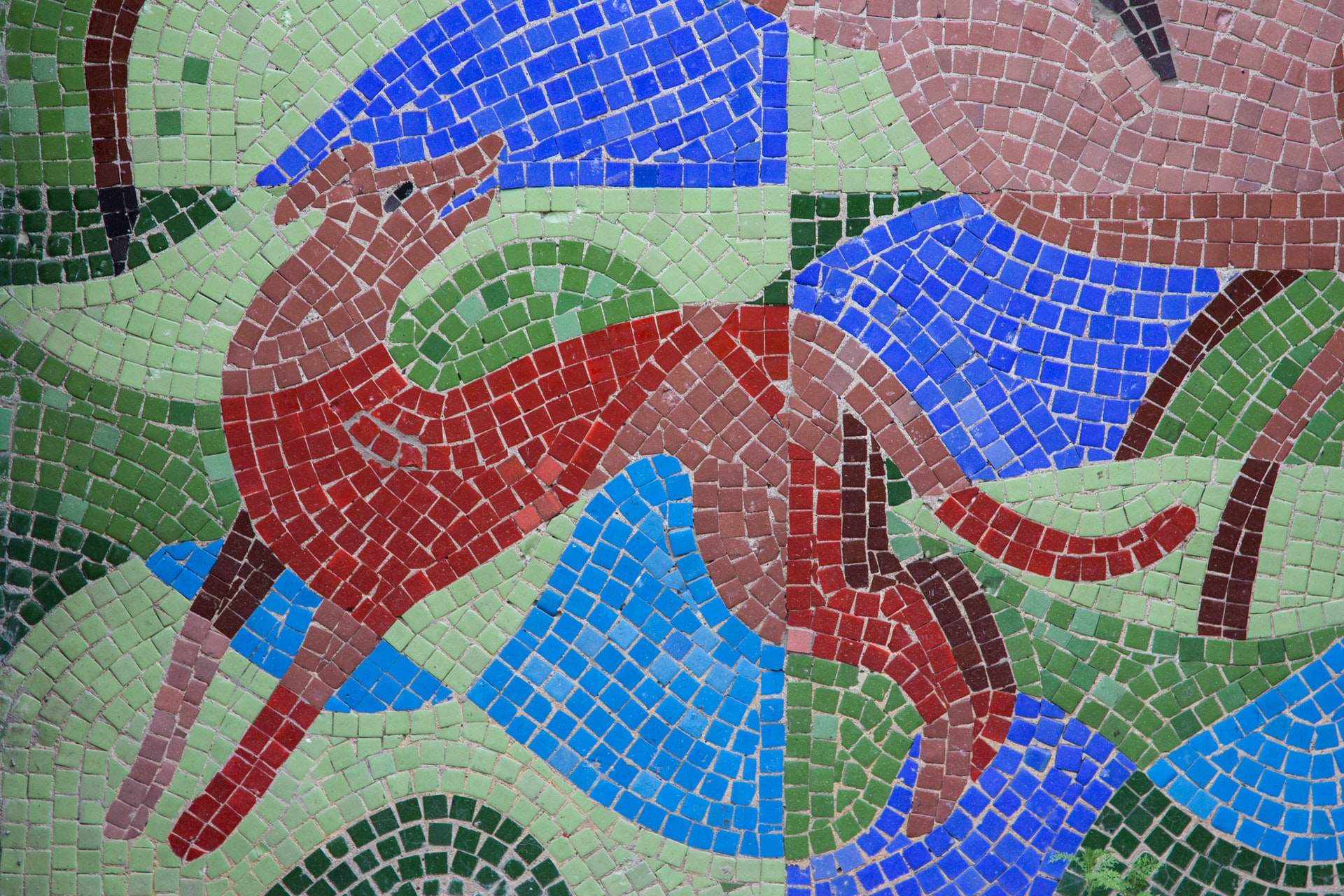
Fragments in React
React components can only return one element. Often, too often, I see developers who therefore enclose their component in a div
. But let me just point out the dangers of this.
The problem
Suppose we have two components, one that creates a table row and one that calls the first to create the actual table. The code will then look something like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
class Table extends Component { render() { return ( <table> <tr> <TableRowData /> </tr> </table> ); } } class TableRowData extends Component { render() { return ( <div> <td>First column</td> <td>Second column</td> </div> ); } }
If we now look at the result, we quickly see what went wrong.
1 2 3 4 5 6 7 8
<table> <tr> <div> <td>First column</td> <td>Second column</td> </div> </tr> </table>
In the middle of the table row (tr
) there is now a div
element, which is of course invalid HTML! Fortunately, React has a solution for this: Fragments.
The solution
Fragments are nothing more than elements that are grouping other elements. The difference with a div
is that the former doesn't add extra nodes to the DOM, causing no impact on the HTML itself. If we rewrite the example above with fragments, we get the following:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
class Table extends Component { render() { return ( <table> <tr> <TableRowData /> </tr> </table> ); } } class TableRowData extends Component { render() { return ( <td>First column</td> <td>Second column</td> ); } }
1 2 3 4 5 6
<table> <tr> <td>First column</td> <td>Second column</td> </tr> </table>
Note, it is certainly not always wrong to return a div
. You can also ask yourself why we want to "pollute" our DOM with extra HTML nodes if they have no added value.
Also nice to know is that fragments have a short syntax, namely empty tags. You can therefore easily replace it in <>
and <
./
>