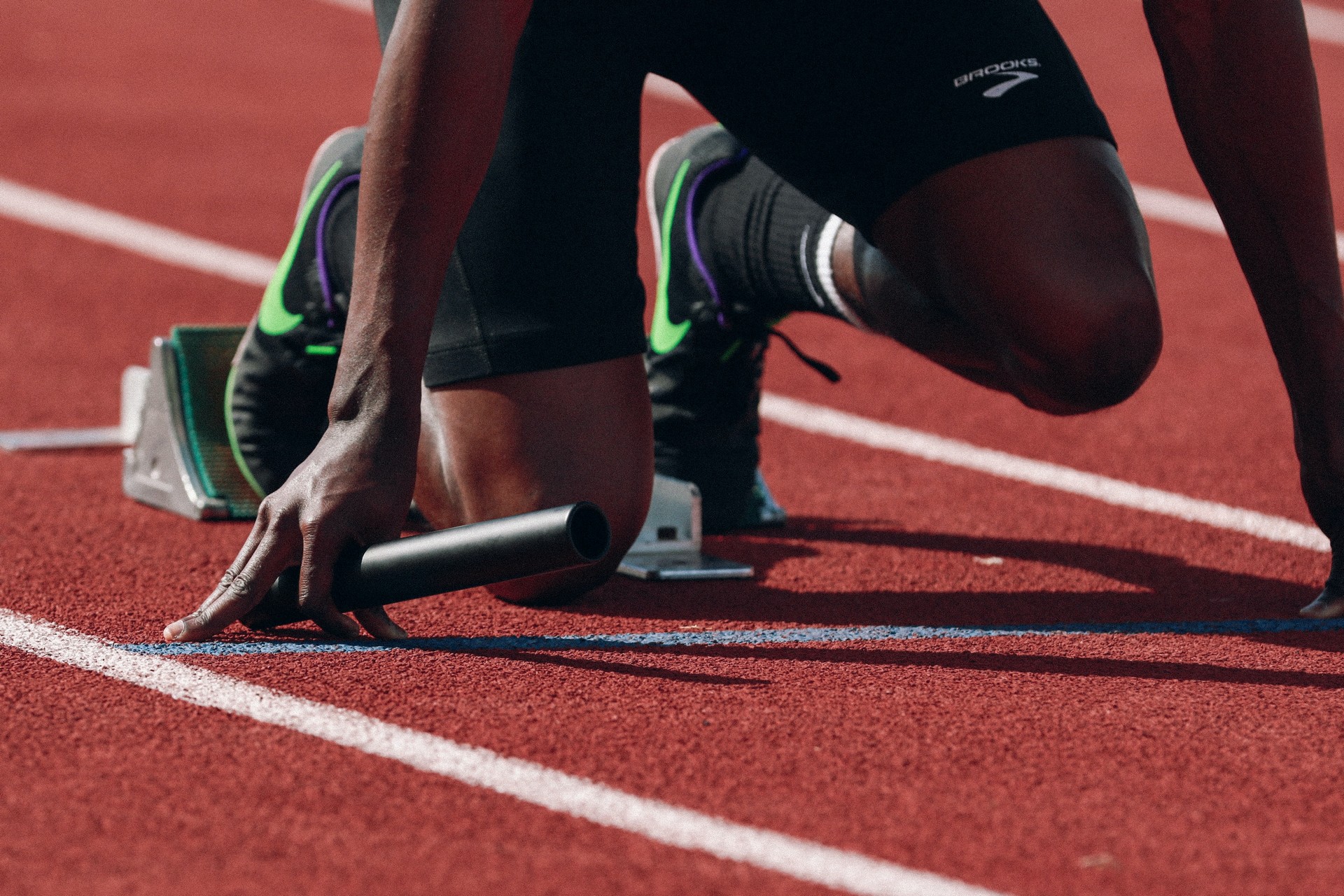
React: Don'ts about props
In React we use props
to pass properties to component. Useful, but you can easily implement them wrong. Below I will discuss two common mistakes.
Props and initial state
It may be tempting to save props directly into state
so they can be modified later. However, be aware that the constructor
is called only once, when a component is created. This means that when a prop changes and the component is called a second time – think of a blog post where you enter the ID of the post in a prop – it does not change the state. Consequently, the earlier data remains visible.
So you may never store props in state
? Only for properties that you only need on a first render and the value of which you then only control from this component itself. In other cases you should fall back to componentDidUpdate()
(in stateful components) of useEffect()
(in stateless components). This lifecycle method (or Hook, in stateless components) allows you to update the component when something, such as props, is changed. Note that componentDidUpdate()
is not called on the first render, only on updates. So make sure you still fill state
with the necessary values from the props.
Key props and indexes
One of the best practices imposed by React itself is to provide a key
prop in dynamic lists of unique value. For your convenience, when building a list of components / elements you can choose to fill that key
with the index of the array element, as shown in an example below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
var data = [ { name: 'T-shirt (male)', serial: '381654' }, { name: 'T-shirt (female)', serial: '654729' }, { name: 'T-shirt (unisex)', serial: '729381' } ] data.map((item, i) => <li key={i}>{item.name}</li> );
At first glance, there is nothing wrong with the above code. Moreover, there isn't even nothing wrong and everything works perfectly. But if we delve deeper into what the key
is used for, the catch will quickly emerge. This prop is used by React to check which items from this list have been changed, added or removed. In the example above, when we change a value in our array, it is impossible for the framework to know which item we have modified and so all elements will have to render again – unnecessarily.
Hence it is recommended to use a stable key
, for example a serial number or other unique value. This way, elements are not unnecessarily re-rendered and you have no impact on performance. The above example is therefore easy to adjust:
1 2 3
data.map((item, i) => <li key={item.serial}>{item.name}</li> );