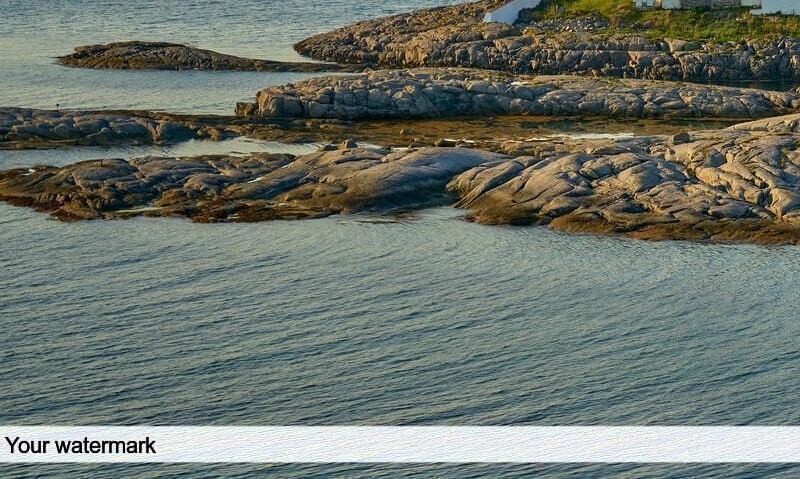
Watermarking images
You may have a collection of photos that you want to exhibit online or forward to third parties. If you want to make sure that the images cannot be claimed by others, you can simply add a watermark. However, this is a lot of work manually, especially with large numbers, so let's see how to do this via a script.
ImageMagick
ImageMagick is a tool to create, edit and convert images. So there are a lot of possibilities, but in this blog post I will limit myself to adding a watermark to a collection of images. The tool can be downloaded for free from the official website.
There are four important commands for what we want to achieve: -fill
, -draw
, -pointsize
and -annotate
.
-fill
: specify the color that ImageMagick should work with to draw or write something – this can be a name, but also a hexadecimal value or an RGB value;-draw
: with this you draw a certain shape on top of the image (such as rectangles or circles);-pointsize
: specify the font size;-annotate
: the easiest way to add text on an image.
Just throw these commands together and we get the following command. Note that the value #ffffff33
is used so the background will be somewhat transparent.
1 2 3 4 5 6
'C:\Program Files\ImageMagick-7.0.10-Q16\magick.exe' convert input.jpg ` -fill #ffffff33 ` -draw "rectangle 0,1010 1920,1050" ` -fill #000000 ` -pointsize 22 ` -annotate +1046 $text "output.jpg"
Of course, our collection of images doesn't consist of photos of all the same dimensions – for starters, some are portrait, some are landscape. The PowerShell function below calculates where the watermark should be positioned, so that it always ends up at the bottom of the image. Feel free to play with the colors and the positions yourself to see the effect.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
[void] [System.Reflection.Assembly]::LoadWithPartialName("System.Drawing") function Add-Watermark($file, $bg, $color, $margin, $size, $text) { $filePath = $file.FullName $fileName = $file.Name $folder = $file.Directory.FullName $img = [Drawing.Image]::FromFile($file.FullName) $w = $img.Width $h = $img.Height $wmh = $size * 1.6 $y = $wmh * 1.5 $rectY1 = $h - $y $rectY2 = $h - $y + $wmh $ty = $h - $y + $margin + $size Write-Host $fileName & 'C:\Program Files\ImageMagick-7.0.10-Q16\magick.exe' convert $filePath ` -fill $bg ` -draw "rectangle 0,${rectY1} ${w},${rectY2}" ` -fill $color ` -pointsize $size ` -annotate +$margin+$ty $text "${folder}\..\output\${fileName}" }
Now simply run this function for your images and they will all be watermarked in no time.