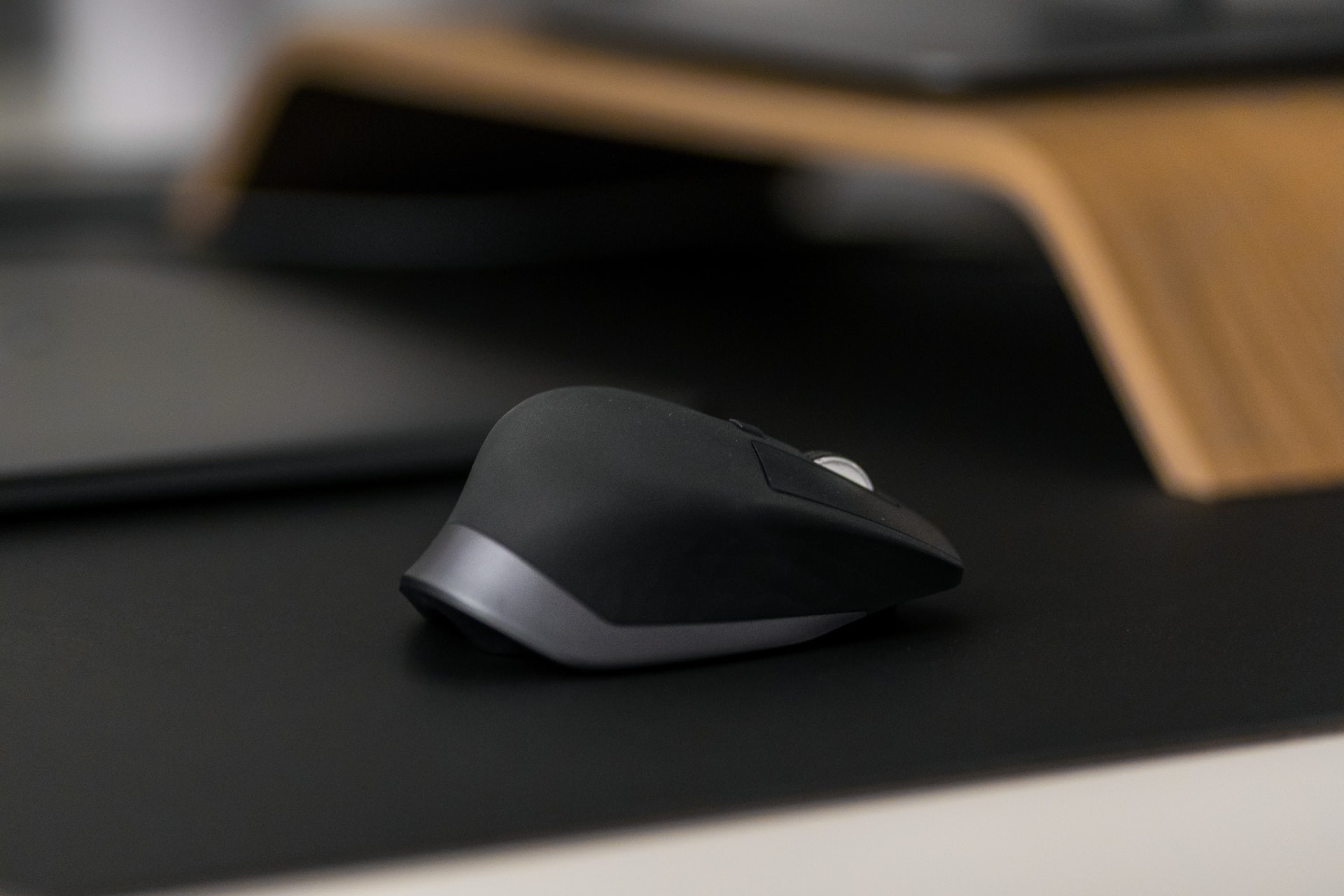
Swapping mouse buttons
I am a lefty. A real one. One who operates his computer mouse with his left hand. One who is also very happy with the Windows setting to toggle the primary mouse button so that I, just like right-handed people, can click with my index finger. A great setting, but one that can annoy many other human beings.
Colleagues who – pre-COVID at least – physically take over my computer can be warned, but even then the context menus are flying around. The worst part is when those same people log into a virtual machine I was last working on. Admittedly, the setting cannot be changed in a flash, but today I finally found a handy solution. Or two, depending on how you agree.
Change the registry
The first of these options is to change the setting directly in the registry. The advantage of this is that you can execute this via a command in a batch file. Once the file has been created, a double click is sufficient – with your primary mouse button of course. The command looks like this:
1
REG ADD "HKCU\Control Panel\Mouse" /t REG_SZ /v SwapMouseButtons /d 1 /f
The above rule sets the mouse buttons for left-handers like me, with a toggled primary button. If you want to reset it, run the same rule again, but change the 1
to a 0
.
Note: this way the change only takes effect when the user logs in again. So you must always remember to execute the command before signing out. I fear that many discussions can arise, similar with raised toilet seats …
Some lines of C #
A better solution, I believe, is a small executable (.exe file) to switch the mouse buttons directly. It is quite easy to create such a file, it can even be done in notepad. Just save it as SwapMouseButtons.cs afterwards.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
using System; using System.Runtime.InteropServices; class SwapMouseButtons { [DllImport("user32.dll")] public static extern Int32 SwapMouseButton(Int32 fSwap); static void Main(string[] args) { // Try to make the right mouse button primary. int alreadyPrimary = SwapMouseButton(1); // If the meaning of the mouse buttons was reversed previously, // before the function was called, the return value is nonzero. // If the meaning of the mouse buttons was not reversed, the // return value is zero. if (alreadyPrimary != 0) { // Make the left mouse button primary. SwapMouseButton(0); } } }
Now all we need to do is compile this so that we have an executable file. You don't need heavy applications for this either, just one simple command. If necessary, change the .NET version in case another version is installed.
1
"%SystemRoot%\Microsoft.NET\Framework64\v4.0.30319\csc" SwapMouseButtons.cs
After you have saved this command as a shortcut, and possibly assigned a keyboard hotkey, you and your colleagues can easily switch the primary button. An inclusive world is one step closer.
The documentaion of SwapMouseButton can be found here.