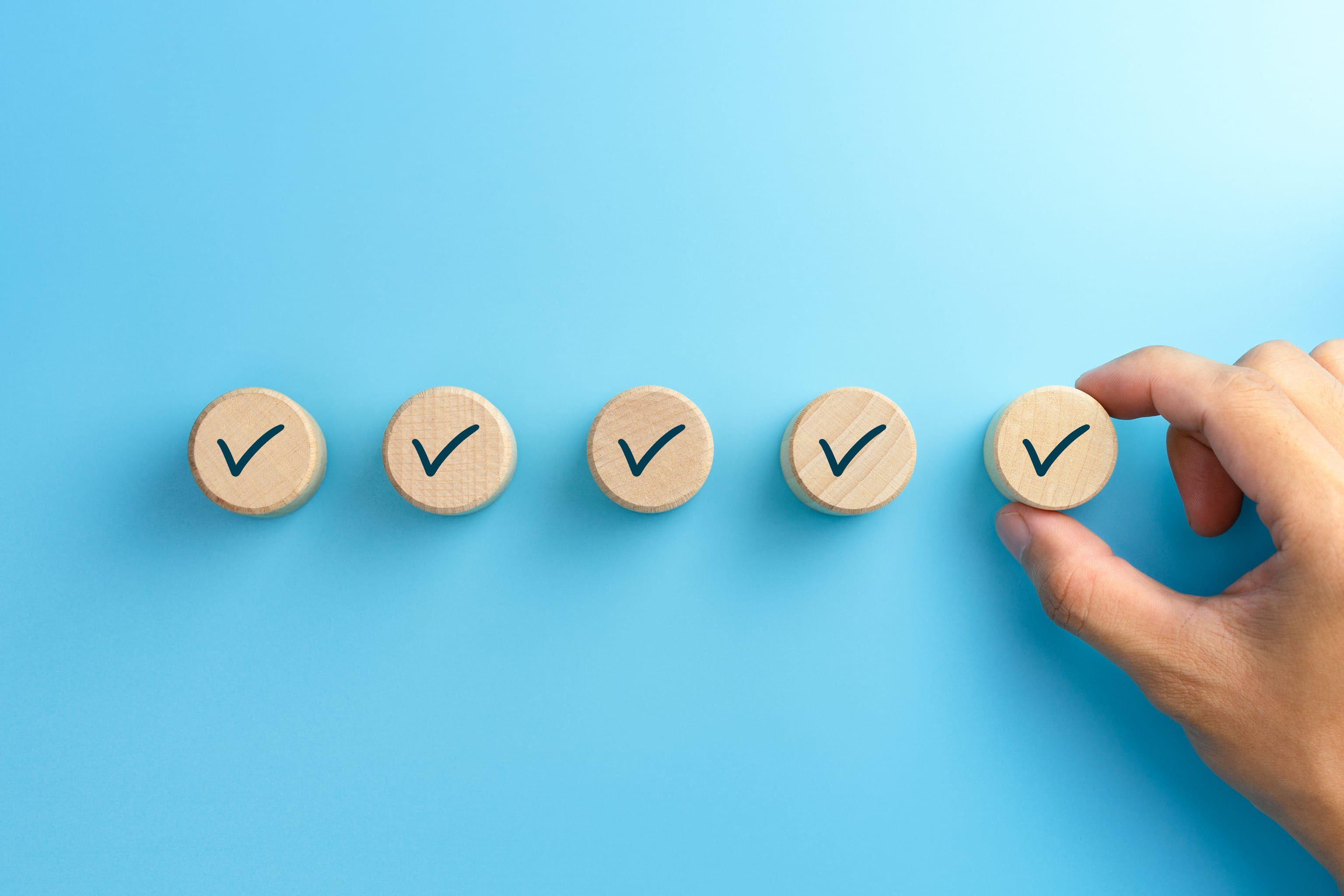
Regular expressions in D365 F&O
A lot of input validation is already handled by Dynamics 365 itself, such as checking whether a number contains no letters. Yet sooner or later you will come to a time where very specific (text) validation is required. In this post I am using the example email addresses.
Regex
We could split the value using string operations to see if the input contains an "@" and a period – in that order – and if there are only alphanumeric characters with dashes if necessary. Not only will this be a cumbersome piece of code, it will also not improve performance and there is a good chance that an error will creep in.
With the help of regular expressions (also called regex) we can do this much shorter, faster and – if we keep our wits about it – also more easily error-free. The only drawback, testing these expressions is not in standard x ++ code. But don't worry, because as you know we can easily access .net from x ++.
Below you can see a runnable class that will test a given value using a regular expression.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
class RegexTester { public static void main(Args _args) { str email = 'info@example.com'; System.Text.RegularExpressions.Regex regex; regex = new System.Text.RegularExpressions.Regex(@'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$'); System.Text.RegularExpressions.Match match; match = regex.Match(email); boolean ret; ret = match.get_Success(); info(strFmt('"%1" is %2 e-mail address.', email, ret ? 'a valid' : 'an invalid')); } }
This way you can quickly and easily perform complex string validations.