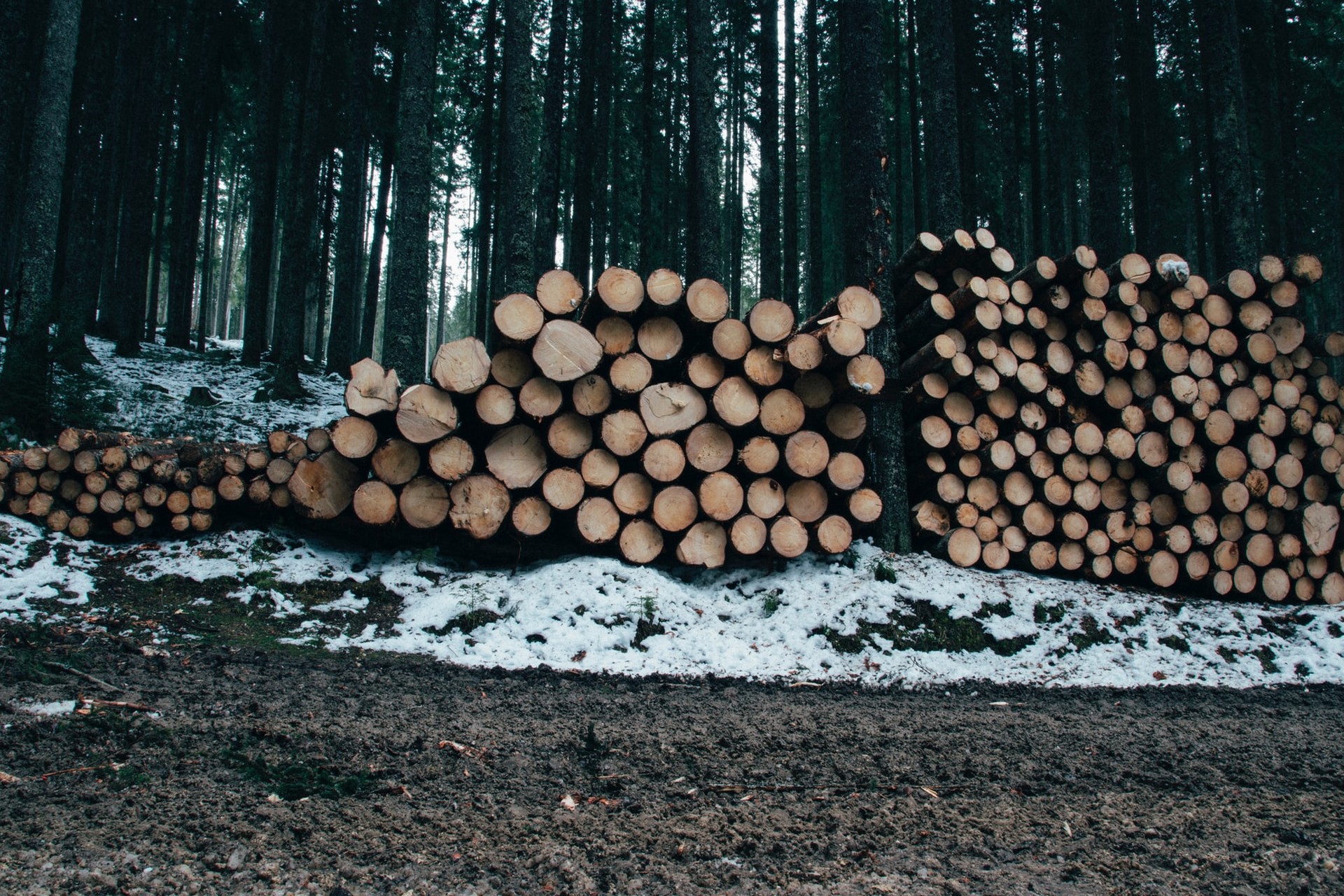
Shorthand logging in JavaScript
It often happens that we use the JavaScript console for debugging and want to log variables. However, I noticed that a lot of people are not yet aware of how we can do this in a fast way, with a shortened notation.
Imagine we have a variable artist
. To write this to the console the following is sufficient.
1
console.log(artist);
The output of this line could then be, for example, "David Bowie". You will of course notice that this is only a handy way if you only want to view one variable or if you can easily distinguish between different variables – for example a string and a number. So we want a way to see the name of the variable as well, not just the value.
It is then that I often see constructs where strings are concatenated or multiple lines are logged to the console. Some examples:
1 2 3 4 5 6 7 8 9
console.log('artist = ' + artist); // artist = David Bowie console.log('artist =', artist); // artist = David Bowie console.log('artist ='); console.log(artist); // artist = // David Bowie
Object logging
I can already hear you thinking: "The goal of this blog post was to find a shorter way, but the above lines are just longer?" To make this shorter, we can use the ability to write entire objects to the console. We don't have to declare new variables for that, we just create the object the moment we want to log it – similar to passing parameters in functions.
1 2
console.log({artist}); //{artist: "David Bowie"}
By only passing the variable to an object, the name of the variable is used as a key. Behind the scenes the following actually happens:
1 2 3 4 5 6 7 8
var artist = 'David Bowie'; var output = { 'artist': artist } console.log(output); //{artist: "David Bowie"}
Both log lines are identical and so we now can very easily write a variable where we can see both the name and the value. An additional advantage is that we can also write other objects in this way. Take a look in your DevTools what you get when you do the following:
1 2 3 4 5 6
var track = { title: 'Heroes', artist: 'David Bowie' } console.log({track})